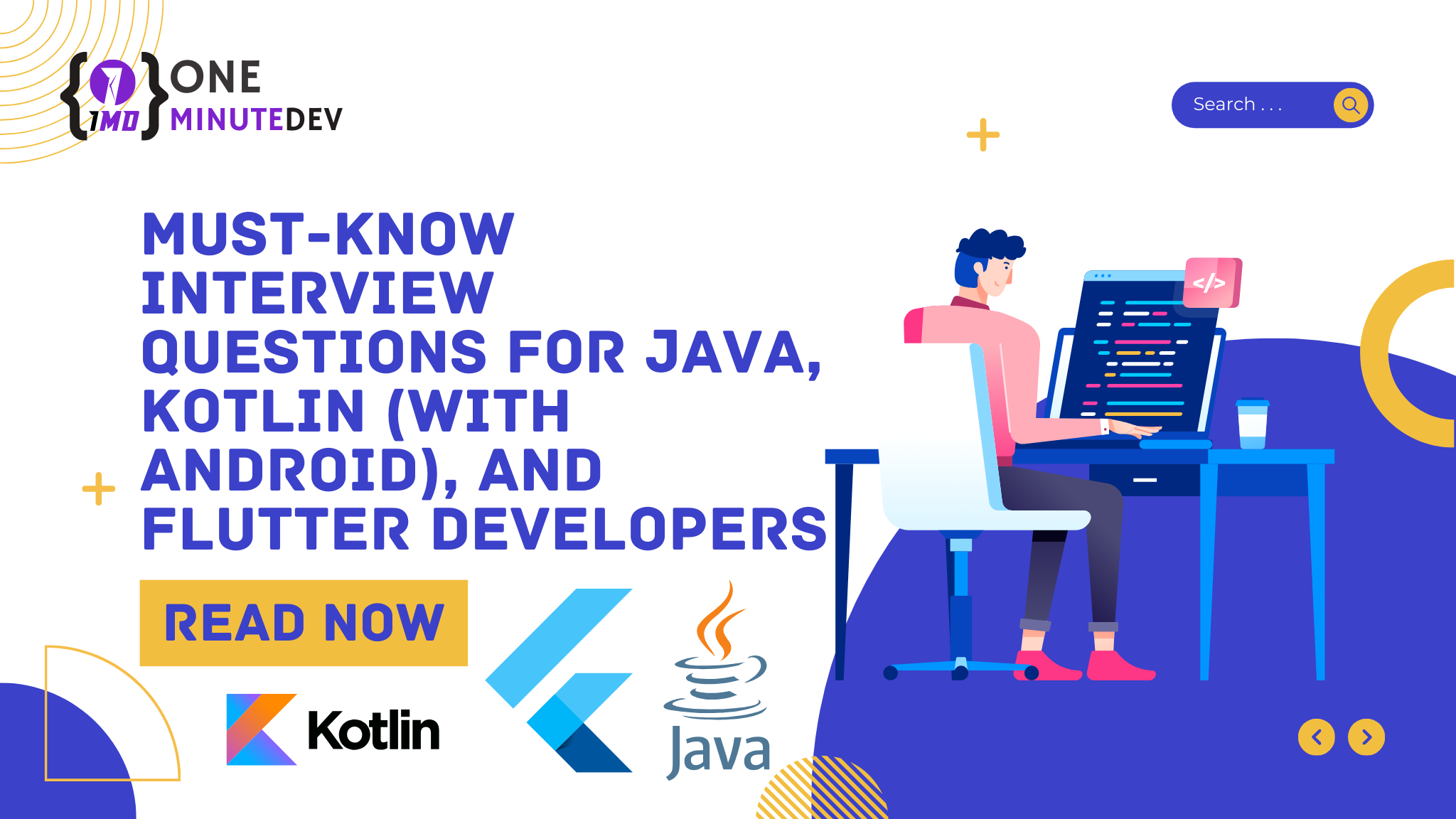
Java
1. What is OOP?
- Answer: Object-Oriented Programming (OOP) is a programming paradigm where programs are organized as a collection of objects. Each object represents an instance of a class.
2. What is Abstraction in OOP?
- Answer: Abstraction is a technique of hiding unnecessary details and showing only essential information. In Java, abstraction can be implemented through abstract classes or interfaces.
3. What is Encapsulation?
- Answer: Encapsulation is the process of wrapping data and methods together into a single unit, like a capsule. In Java, setter and getter methods are used to access the encapsulated data, with Java Beans being a classic example of a fully encapsulated class.
4. What is Inheritance?
- Answer: Inheritance is a mechanism in OOP where one class acquires properties and behaviors from a parent class, enabling code reusability.
5. What is Polymorphism?
- Answer: Polymorphism is an OOP concept where one action can be performed in different ways. Java supports polymorphism through method overloading and method overriding.
6. Why is Java considered portable?
- Answer: Java is portable because it compiles code into bytecode, which can run on any operating system with a Java Virtual Machine (JVM) without modification.
7. What are the advantages of Java?
- Answer:
- Simple and easy to learn.
- Object-oriented, allowing for reusable code.
- Platform-independent due to its bytecode.
- Supports distributed computing.
- Secure, with built-in security features.
- Efficient memory allocation and garbage collection.
- Multithreaded, allowing multiple threads to run concurrently.
8. Why doesn’t Java support multiple inheritance, but C++ does?
- Answer: Java does not support multiple inheritance to avoid complexity and ambiguity that arise with it, such as the “Diamond Problem.” Instead, Java uses interfaces to achieve similar functionality. C++ supports multiple inheritance but requires careful management to avoid conflicts.
9. What’s the difference between an abstract class and an interface?
- Answer:
- An abstract class can have both abstract and non-abstract methods, while an interface can have only abstract methods (in Java 8 and above, interfaces can have default methods).
- An abstract class uses the
extends
keyword, while an interface is implemented withimplements
. - An abstract class can implement an interface, but an interface cannot extend an abstract class.
10. Difference between final
, finally
, and finalize
:
- Answer:
final
: A keyword to restrict class, method, or variable usage. A final class cannot be subclassed, a final method cannot be overridden, and a final variable’s value cannot change.finally
: A block used in exception handling to execute important code regardless of exception occurrence.finalize
: A method that allows for clean-up operations before an object is garbage-collected.
11. Difference between final
and const
:
- Answer:
const
is used to declare constant variables, but in Java,const
is not a keyword. Instead,final
is used to make variables constant and unchangeable.
12. How can a single variable be used by two threads, but not simultaneously?
- Answer: Using the
volatile
keyword ensures that the variable is accessed by only one thread at a time.volatile
prevents the compiler from making optimizations that might interfere with multi-threaded operations on that variable.
13. Difference between StringBuilder
and StringBuffer
:
- Answer:
StringBuffer
is synchronized and thread-safe, meaning two threads cannot access its methods simultaneously.StringBuilder
is non-synchronized and not thread-safe, so two threads can call its methods concurrently, which makes it faster thanStringBuffer
in single-threaded scenarios.
Kotlin
1. What are the advantages of Kotlin?
- Answer: Kotlin offers faster compilation, efficient deployment, and is lightweight, which helps in preventing applications from increasing in size. It is designed for seamless interoperability with Java and provides concise syntax, null safety, and functional programming support.
2. What is the difference between val
and var
in Kotlin?
- Answer:
var
: A mutable variable that can be reassigned multiple times.val
: An immutable variable that can be assigned only once and cannot be changed after initialization.
3. What is the difference between val
and const
in Kotlin?
- Answer:
const
: A compile-time constant whose value is known at compile time.val
: A runtime constant whose value is determined at runtime.
4. Can we make a class final in Kotlin?
- Answer: In Kotlin, classes are
final
by default, meaning they cannot be inherited. To allow inheritance, theopen
keyword must be used.
5. What is inheritance in Kotlin?
- Answer: Inheritance allows a class to inherit properties and methods from another class. In Kotlin, a class must be marked as
open
to be inheritable.
6. Difference between safe call ?
and null check !!
operators in Kotlin:
- Answer:
?
: The safe call operator is used when a variable may be null, and it safely returns null if the variable is null.!!
: The non-null assertion operator throws aNullPointerException
if the variable is null, asserting that the variable is never null.
7. What are Kotlin Coroutines?
- Answer: Coroutines are a feature in Kotlin that enables asynchronous programming by converting long-running tasks (like database or network calls) into sequential code, making it easier to write and maintain asynchronous code.
8. What is an Extension Function in Kotlin?
- Answer: Extension functions allow adding new methods to classes without inheriting from them or using design patterns. They are defined with the syntax
fun <class_name>.<method_name>()
.
9. What is a Label in Kotlin?
- Answer: In Kotlin, any expression can be marked with a label, which helps identify and control flow statements like
break
andcontinue
within nested loops or expressions.
10. What are Scope Functions in Kotlin?
- Answer: Scope functions in Kotlin (like
let
,run
,with
,apply
, andalso
) execute a block of code within the context of an object, simplifying code by reducing boilerplate when working with that object.
Android
1. What is the Activity Lifecycle in Android?
- Answer: The activity lifecycle defines how activities transition between states in response to user interactions or system events. Key lifecycle methods include:
onCreate()
: Called when the activity is first created.onStart()
: Called when the activity becomes visible.onResume()
: Called when the activity starts interacting with the user.onPause()
: Called when the activity is partially obscured.onStop()
: Called when the activity is no longer visible.onDestroy()
: Called before the activity is destroyed.
2. What is the Fragment Lifecycle in Android?
- Answer: Fragments follow a lifecycle that helps manage their UI and interactions within an activity:
onAttach
,onCreate
,onCreateView
,onActivityCreate
,onStart
,onResume
,onPause
,onStop
,onDestroyView
,onDestroy
,onDetach
3. What is the Android Architecture?
- Answer: The Android architecture consists of several layers:
- Applications: The top layer where user applications run.
- Application Framework: Provides APIs for managing application components.
- Android Runtime and Platform Libraries: Libraries and the Android Runtime (ART) that power Android apps.
- Linux Kernel: The foundation providing low-level system functionalities.
4. What is a Fragment in Android?
- Answer: A fragment is a modular section of an activity, used to create more flexible and reusable UI components.
5. How to Clear All Fragments in Backstack and Go to a New Fragment?
- Answer:
FragmentManager fm = getActivity().getSupportFragmentManager();
for (int i = 0; i < fm.getBackStackEntryCount(); ++i) {
fm.popBackStack();
}
6. How to Access a Parent Fragment’s Method in a Child Fragment?
- Answer: You can use
parentFragment.methodName()
to access a method of the parent fragment from the child fragment.
7. What are ViewBinding and DataBinding?
- Answer:
- ViewBinding: Binds views directly to the code.
- DataBinding: Binds data to views, allowing for two-way data binding alongside view binding.
8. What is a Service in Android?
- Answer: A service is an application component that performs long-running operations in the background without a UI.
9. What is IntentService in Android?
- Answer:
IntentService
is a base class for services that handle asynchronous requests (expressed as intents) in the background.
10. What is Gradle?
- Answer: Gradle is an open-source build automation tool used for building, testing, and deploying Android applications.
11. What is the Android Manifest?
- Answer: The Android manifest file contains essential information about the app, including permissions, components, and application metadata, necessary for Android to execute the app.
12. What is Jetpack?
- Answer: Jetpack is a suite of libraries, tools, and guidance provided by Google to build high-quality Android apps. Jetpack Compose is a part of Jetpack, offering modern UI design for native Android applications.
13. What is Dependency Injection (DI)?
- Answer: Dependency Injection is a design pattern used to manage dependencies by injecting them where needed, promoting a modular and testable app architecture.
14. What are Room Database, LiveData, and ViewModel?
- Answer:
- Room Database: Provides an abstraction layer over SQLite to allow database access while leveraging the full power of SQLite.
- LiveData: An observable data holder that updates the UI in response to data changes.
- ViewModel: Manages UI-related data in a lifecycle-conscious way, separating the data from the UI controller logic.
15. SQL Query: Get the Employee with the Maximum Salary
- Answer:
sql SELECT * FROM employee ORDER BY salary DESC LIMIT 1;
16. SQL Query: Get the Employee with the Maximum Salary from Two Tables (employee
and salary
)
- Answer:
sql SELECT * FROM employee, salary WHERE employee.id = salary.emp_id ORDER BY salary DESC LIMIT 1;
17. What are Launch Modes in Android?
- Answer:
standard
: A new instance is created each time.singleTop
: If an instance is already at the top, it is reused; otherwise, a new instance is created.singleTask
: A new task is created and only one instance of the activity exists.singleInstance
: The activity has its task with only one instance.
18. What is a Content Provider in Android?
- Answer: A content provider manages shared app data, allowing other applications to securely access and modify that data.
19. What is an Intent and its Types?
- Answer:
- Explicit Intent: Specifies the exact component (activity or service) to start.
- Implicit Intent: Specifies an action to be performed, allowing other apps to respond.
20. What is AIDL (Android Interface Definition Language)?
- Answer: AIDL is a language used to define the interface for communication between different processes in Android, enabling inter-process communication (IPC).
Flutter
1. What is Flutter?
- Answer: Flutter is Google’s UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase. It uses the Dart programming language and provides fast and expressive UIs with customizable widgets.
2. What are the Advantages of Flutter?
- Answer:
- Same UI and business logic across platforms.
- Reduced code development time.
- Faster time-to-market.
- Near-native app performance.
- Support for custom, complex animated UIs.
- Own rendering engine for cross-platform consistency.
- Simple platform-specific logic integration.
- Potential to extend beyond mobile platforms (e.g., web, desktop).
3. Why Use Dart in Flutter?
- Answer: Flutter uses Dart because it enables Flutter to avoid needing a separate declarative layout language like JSX or XML. Dart’s compilation to native code also improves performance and helps Flutter handle asynchronous operations efficiently.
4. What is the Difference Between Stateless and Stateful Widgets?
- Answer:
- Stateless Widget: UI that does not change once built. The
build
function is only called once. - Stateful Widget: UI that can change or update its state after it is built. The widget maintains a state object, allowing it to be altered.
- Stateless Widget: UI that does not change once built. The
5. Difference Between Expanded and Flexible in Flutter:
- Answer:
- Flexible: By default, wraps content, but you can adjust the size using the
Fit
parameter. - Expanded: Forces the child widget to take up all available space (match-parent behavior), with adjustments possible using
flex
.
- Flexible: By default, wraps content, but you can adjust the size using the
6. What is a Stack in Flutter?
- Answer:
Stack
is a widget that allows positioning its children relative to the edges of its container, enabling layered UI layouts.
7. What is the Lifecycle of a Stateful Widget in Flutter?
- Answer: The lifecycle of a stateful widget includes:
createState()
: Creates the mutable state.initState()
: Initializes the widget state.didChangeDependencies()
: Called when widget dependencies change.build()
: Builds the UI.didUpdateWidget(Widget oldWidget)
: Called when the widget is rebuilt.setState()
: Triggers a rebuild with updated state.deactivate()
: Called when the widget is removed from the tree.dispose()
: Cleans up resources when the widget is destroyed.
8. What is App State?
- Answer: App state refers to everything stored in memory while the app is running, such as user settings, session information, and data needed to display the UI correctly.
9. How Does Flutter Communicate with Native Code?
- Answer: Flutter communicates with native code using
MethodChannel
, which facilitates calling platform-specific code (e.g., Android or iOS) from Flutter and returning results.
10. What is YAML in Flutter?
- Answer: YAML (Yet Another Markup Language) is used in Flutter’s
pubspec.yaml
file to define metadata for a project, such as package name, version, author, and dependencies. YAML is also used in federated plugins to separate platform-specific code.
11. What Options are Available for Local Storage in Flutter?
SharedPreferences: Stores key-value pairs for small amounts of persistent data.
Answer:
SQLite: Provides a local SQL database for structured data.
Firebase: Offers cloud-based data storage with synchronization support.
Most Valuable Flutter Interview Questions to Master
Flutter Widget Lifecycle
- createState(): Creates the mutable state for a stateful widget.
- initState(): Called once when the widget is inserted.
- didChangeDependencies(): Called after
initState
. - build(): Builds the widget’s UI.
- didUpdateWidget(): Called when the parent widget changes.
- setState(): Triggers a rebuild.
- deactivate(): Called when the widget is removed from the tree.
- dispose(): Cleans up resources when the widget is destroyed.
Flutter State Management
- Provider: Simplifies state management with a central place to store state.
- BLoC: Uses streams and reactive programming to separate business logic.
- MobX: A reactive state management library.
- Riverpod: A more robust alternative to Provider.
- InheritedWidget: Passes data to descendant widgets in the tree.
- Redux: Good for large applications with a global state container.
Streams in Dart
- Single Subscription Streams: Allows only one listener; data must be received in sequence.
- Broadcast Streams: Allows multiple listeners for independent events.
Expanded vs. Flexible Widgets
- Flexible: Wraps content, with optional resizing using
Fit
. - Expanded: Fills available space (similar to
match_parent
).
Flutter Lifecycle: didChangeDependencies()
vs didUpdateWidget()
didChangeDependencies()
: Called afterinitState()
, typically used when dependencies change.didUpdateWidget()
: Called when the parent widget changes, requiring a UI redraw.
Null-Aware Operators in Dart
??
: Provides a default if the variable is null (e.g.,var x = y ?? 2
).??=
: Assigns a value only if the variable is null (e.g.,x ??= 1
).
Mixins in Flutter
Mixins allow code reuse without requiring inheritance, useful in Dart for implementing multiple behaviors.
Yield and Yield* in BLoC
yield
: Adds a value to a stream in an async function, similar to a return but continues the function.yield*
: Delegates control to another generator function temporarily.
Tree Shaking in Flutter
Tree shaking removes unused code from the compiled app, reducing size by excluding dead code.
WidgetsApp vs. MaterialApp
- WidgetsApp: Basic app setup, handling essentials like the system back button.
- MaterialApp: Builds on WidgetsApp with material design features like theming.
Testing in Flutter
- Unit Tests: Tests business logic.
- Widget Tests: Tests UI components.
- Integration Tests: Tests the app end-to-end.
LayoutBuilder in Flutter
Provides constraints of the parent widget, helpful when sizing depends on the parent rather than the child.
Factory Constructors in Dart
A factory constructor returns an instance of a class without necessarily creating a new instance each time.
Example:
class Car {
String make;
String model;
String yearMade;
bool hasABS;
factory Car.ford(String model, String yearMade, bool hasABS) {
return FordCar(model, yearMade, hasABS);
}
}
class FordCar extends Car {
FordCar(String model, String yearMade, bool hasABS)
: super("Ford", model, yearMade, hasABS);
}
Keys in Flutter
- GlobalKey: Unique across the app, useful for accessing a widget outside its subtree.
- UniqueKey: Unique within a widget’s parent, useful in lists.
- ValueKey: Based on a value, good for identifying a widget within a list based on a unique property.
Ticker in Flutter
A Ticker generates signals at regular intervals, used to drive animations at around 60 frames per second.
Logical Questions and Flutter/Dart Concepts Explained
Logical Question: Swap Values Without Using a Third Variable or +
and -
Operators For a = 14
and b = 12
, we can swap values by using bitwise XOR:
int a = 14;
int b = 12;
a = a ^ b; // a becomes 26 (14 XOR 12)
b = a ^ b; // b becomes 14 (26 XOR 12)
a = a ^ b; // a becomes 12 (26 XOR 14)
print("a = $a, b = $b"); // Output: a = 12, b = 14